Androidの模倣QQのログインインターフェイスの例、ログインと登録機能を実現するために。
2022-02-18 18:36:42
最初の文
私は、以下の私の公開ウェブサイトをフォローすることを歓迎します。 矢口ジュニア
WeChat公開サイトでは、優先的に記事を更新しています。上のQRコードをスキャンしてフォローしてください 共に進み、共に育つ。
Androidの開発では、登録やログインの機能を使うことが多いので、一般的なログインのインターフェースをまとめて、それに対応する機能を実装しました。ご参考までに。このプロジェクトでは、ログイン、登録画面、パスワード取得の3つのアクティビティが含まれています。
GitHubのソースコードのアドレスです。 ログインテスト
インターフェース
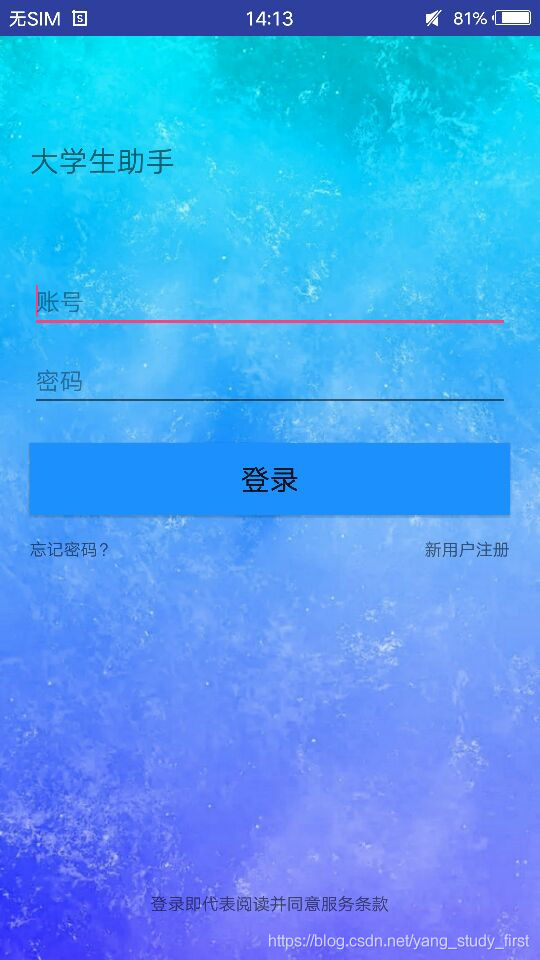
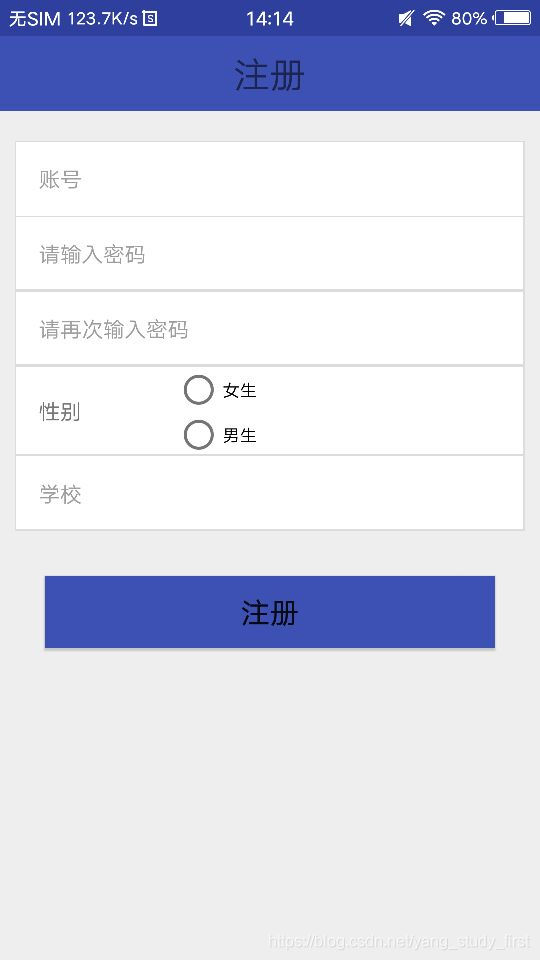
コードがどのようにパースされたかを示します。
まず、ログイン画面のactivity_main.xmlです。
public class MainActivity extends AppCompatActivity {
private String userName,psw,spPsw;//get username, password, encrypted password
private EditText et_user_name,et_psw;//edit box
@Override
protected void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// set this interface to vertical
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
init();
}
//Get the interface controls
private void init() {
//get the id from main_title_bar
//from activity_login.xml
TextView tv_register = (TextView) findViewById(R.id.tv_register);
TextView tv_find_psw = (TextView) findViewById(R.id.tv_find_psw);
Button btn_login = (Button) findViewById(R.id.btn_login);
et_user_name= (EditText) findViewById(R.id.et_user_name);
et_psw= (EditText) findViewById(R.id.et_psw);
// Immediately register the control's click event
tv_register.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// In order to jump to the registration screen and implement the registration function
Intent intent=new Intent(MainActivity.this,RegisterActivity.class);
startActivityForResult(intent, 1);
}
});
//click event for password retrieval control
tv_find_psw.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this,LostFindActivity.class));
}
});
//click event of login button
btn_login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//start login, get user name and password getText().toString().trim();
userName = et_user_name.getText().toString().trim();
psw = et_psw.getText().toString().trim();
// the current user input password for MD5 encryption and then comparison judgment, MD5Utils.md5( ); psw encryption to determine whether the same
String md5Psw = MD5Utils.md5(psw);
// md5Psw ; spPsw reads the password based on the username from SharedPreferences
// Define the method readPsw in order to read the user name and get the password
spPsw = readPsw(userName);
// TextUtils.isEmpty
if (TextUtils.isEmpty(userName)) {
Toast.makeText(MainActivity.this, "Please enter user name", Toast.LENGTH_SHORT).show();
} else if (TextUtils.isEmpty(psw)) {
Toast.makeText(MainActivity.this, "Please enter your password", Toast.LENGTH_SHORT).show();
// md5Psw.equals(); determine if the encrypted password is the same as the one saved in SharedPreferences
} else if (md5Psw.equals(spPsw)) {
//consistent login success
Toast.makeText(MainActivity.this, "Login successful", Toast.LENGTH_SHORT).show();
// Save the login status, save the login user name in the interface Define a method saveLoginStatus boolean status , userName username;
saveLoginStatus(true, userName);
//Close this page to the home page after successful login
Intent data = new Intent();
//datad.putExtra( ); name , value ;
data.putExtra("isLogin", true);
// RESULT_OK is an Activity system constant with a status code of -1
// indicates that the content of this page will return data to the previous page successfully, if it is returned with back, there is no setResult to pass the data value
setResult(RESULT_OK, data);
//Destroy the login screen
MainActivity.this.finish();
//jump to the main interface and pass the status of successful login to the MainActivity
startActivity(new Intent(MainActivity.this, ItemActivity.class));
} else if ((spPsw ! = null && !TextUtils.isEmpty(spPsw) && !md5Psw.equals(spPsw))) {
Toast.makeText(MainActivity.this, "The username and password entered do not match", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(MainActivity.this, "This username does not exist", Toast.LENGTH_SHORT).show();
}
}
});
}
/**
* Read password from SharedPreferences based on username
*/
private String readPsw(String userName){
//getSharedPreferences("loginInfo",MODE_PRIVATE);
public class RegisterActivity extends AppCompatActivity {
// controls for username, password, re-entered password
private EditText et_user_name,et_psw,et_psw_again;
//get the values of the controls for user name, password and re-entered password
private String userName,psw,pswAgain;
private RadioGroup Sex;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//set the page layout ,register the interface
setContentView(R.layout.activity_register);
//set this interface to vertical screen
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
init();
}
private void init() {
//get the corresponding UI control from the activity_register.xml page
Button btn_register = (Button) findViewById(R.id.btn_register);
et_user_name= (EditText) findViewById(R.id.et_user_name);
et_psw= (EditText) findViewById(R.id.et_psw);
et_psw_again= (EditText) findViewById(R.id.et_psw_again). et_psw_again= (EditText) findViewById(R.id.et_psw_again);
Sex= (RadioGroup) findViewById(R.id.SexRadio);
//register button
btn_register.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//get the string entered in the corresponding control
getEditString();
//judge the content of the input box
int sex;
int sexChoseId = Sex.getCheckedRadioButtonId();
switch (sexChoseId) {
case R.id.mainRegisterRdBtnFemale:
sex = 0;
break;
case R.id.mainRegisterRdBtnMale:
sex = 1;
break;
default:
sex = -1;
break;
}
if(TextUtils.isEmpty(userName)){
Toast.makeText(RegisterActivity.this, "Please enter user name", Toast.LENGTH_SHORT).show();
}else if(TextUtils.isEmpty(psw)){
Toast.makeText(RegisterActivity.this, "Please enter your password", Toast.LENGTH_SHORT).show();
}else if(TextUtils.isEmpty(pswAgain)) {
Toast.makeText(RegisterActivity.this, "Please enter your password again", Toast.LENGTH_SHORT).show();
} else if (sex<0){
Toast.makeText(RegisterActivity.this, "Please select gender", Toast.LENGTH_SHORT).show();
}else if(!psw.equals(pswAgain)){
Toast.makeText(RegisterActivity.this, "The password entered twice is not the same", Toast.LENGTH_SHORT).show();
/**
* Read the entered username from SharedPreferences and determine if the username is available in SharedPreferences
*/
}else if(isExistUserName(userName)){
Toast.makeText(RegisterActivity.this, "This account name already exists", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(RegisterActivity.this, "Registration successful", Toast.LENGTH_SHORT).show();
//Save the account number, password and account ID to the sp
/**
* Save account number and password to SharedPreferences
*/
saveRegisterInfo(userName, psw);
// pass the account number to LoginActivity.java after successful registration
// return the value to the loginActivity display
Intent data = new Intent();
data.putExtra("userName", userName);
setResult(RESULT_OK, data);
// RESULT_OK is an Activity system constant with a status code of -1.
// indicates that the content operation under this page succeeded in returning the data to the previous page, if it is returned with back then there is no data value passed with setResult
RegisterActivity.this.finish();
}
}
});
}
/**
* Get the string in the control
*/
private void getEditString(){
userName=et_user_name.getText().toString().trim();
psw=et_psw.getText().toString().trim();
pswAgain=et_psw_again.getText().toString().trim();
}
/**
* Read the entered user name from SharedPreferences and determine if the user name is available in SharedPreferences
*/
private boolean isExistUserName(String userName){
boolean has_userName=false;
// mode_private SharedPreferences sp = getSharedPreferences( );
// "loginInfo", MODE_PRIVATE
SharedPreferences sp = getSharedPreferences("loginInfo", MODE_PRIVATE);
//Get the password
String spPsw=sp.getString(userName, "");//pass in the user name to get the password
//If the password is not empty, then the user name was indeed saved
if(!TextUtils.isEmpty(spPsw)) {
has_userName=true;
}
return has_userName;
}
/**
* Save account and password to SharedPreferences SharedPreferences
*/
private void saveRegisterInfo(String userName,String psw){
String md5Psw = MD5Utils.md5(psw);// encrypt the password with MD5
//loginInfo means file name, mode_private SharedPreferences sp = getSharedPreferences( );
SharedPreferences sp=getSharedPreferences("loginInfo", MODE_PRIVATE);
//get the editor, SharedPref
ログインアクティビティMainActivityに対応します。
public class MainActivity extends AppCompatActivity {
private String userName,psw,spPsw;//get username, password, encrypted password
private EditText et_user_name,et_psw;//edit box
@Override
protected void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// set this interface to vertical
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
init();
}
//Get the interface controls
private void init() {
//get the id from main_title_bar
//from activity_login.xml
TextView tv_register = (TextView) findViewById(R.id.tv_register);
TextView tv_find_psw = (TextView) findViewById(R.id.tv_find_psw);
Button btn_login = (Button) findViewById(R.id.btn_login);
et_user_name= (EditText) findViewById(R.id.et_user_name);
et_psw= (EditText) findViewById(R.id.et_psw);
// Immediately register the control's click event
tv_register.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// In order to jump to the registration screen and implement the registration function
Intent intent=new Intent(MainActivity.this,RegisterActivity.class);
startActivityForResult(intent, 1);
}
});
//click event for password retrieval control
tv_find_psw.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(MainActivity.this,LostFindActivity.class));
}
});
//click event of login button
btn_login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//start login, get user name and password getText().toString().trim();
userName = et_user_name.getText().toString().trim();
psw = et_psw.getText().toString().trim();
// the current user input password for MD5 encryption and then comparison judgment, MD5Utils.md5( ); psw encryption to determine whether the same
String md5Psw = MD5Utils.md5(psw);
// md5Psw ; spPsw reads the password based on the username from SharedPreferences
// Define the method readPsw in order to read the user name and get the password
spPsw = readPsw(userName);
// TextUtils.isEmpty
if (TextUtils.isEmpty(userName)) {
Toast.makeText(MainActivity.this, "Please enter user name", Toast.LENGTH_SHORT).show();
} else if (TextUtils.isEmpty(psw)) {
Toast.makeText(MainActivity.this, "Please enter your password", Toast.LENGTH_SHORT).show();
// md5Psw.equals(); determine if the encrypted password is the same as the one saved in SharedPreferences
} else if (md5Psw.equals(spPsw)) {
//consistent login success
Toast.makeText(MainActivity.this, "Login successful", Toast.LENGTH_SHORT).show();
// Save the login status, save the login user name in the interface Define a method saveLoginStatus boolean status , userName username;
saveLoginStatus(true, userName);
//Close this page to the home page after successful login
Intent data = new Intent();
//datad.putExtra( ); name , value ;
data.putExtra("isLogin", true);
// RESULT_OK is an Activity system constant with a status code of -1
// indicates that the content of this page will return data to the previous page successfully, if it is returned with back, there is no setResult to pass the data value
setResult(RESULT_OK, data);
//Destroy the login screen
MainActivity.this.finish();
//jump to the main interface and pass the status of successful login to the MainActivity
startActivity(new Intent(MainActivity.this, ItemActivity.class));
} else if ((spPsw ! = null && !TextUtils.isEmpty(spPsw) && !md5Psw.equals(spPsw))) {
Toast.makeText(MainActivity.this, "The username and password entered do not match", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(MainActivity.this, "This username does not exist", Toast.LENGTH_SHORT).show();
}
}
});
}
/**
* Read password from SharedPreferences based on username
*/
private String readPsw(String userName){
//getSharedPreferences("loginInfo",MODE_PRIVATE);
次に、登録画面 activity_register.xml です。
登録アクティビティRegisterActivityに対応しています。
public class RegisterActivity extends AppCompatActivity {
// controls for username, password, re-entered password
private EditText et_user_name,et_psw,et_psw_again;
//get the values of the controls for user name, password and re-entered password
private String userName,psw,pswAgain;
private RadioGroup Sex;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//set the page layout ,register the interface
setContentView(R.layout.activity_register);
//set this interface to vertical screen
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
init();
}
private void init() {
//get the corresponding UI control from the activity_register.xml page
Button btn_register = (Button) findViewById(R.id.btn_register);
et_user_name= (EditText) findViewById(R.id.et_user_name);
et_psw= (EditText) findViewById(R.id.et_psw);
et_psw_again= (EditText) findViewById(R.id.et_psw_again). et_psw_again= (EditText) findViewById(R.id.et_psw_again);
Sex= (RadioGroup) findViewById(R.id.SexRadio);
//register button
btn_register.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//get the string entered in the corresponding control
getEditString();
//judge the content of the input box
int sex;
int sexChoseId = Sex.getCheckedRadioButtonId();
switch (sexChoseId) {
case R.id.mainRegisterRdBtnFemale:
sex = 0;
break;
case R.id.mainRegisterRdBtnMale:
sex = 1;
break;
default:
sex = -1;
break;
}
if(TextUtils.isEmpty(userName)){
Toast.makeText(RegisterActivity.this, "Please enter user name", Toast.LENGTH_SHORT).show();
}else if(TextUtils.isEmpty(psw)){
Toast.makeText(RegisterActivity.this, "Please enter your password", Toast.LENGTH_SHORT).show();
}else if(TextUtils.isEmpty(pswAgain)) {
Toast.makeText(RegisterActivity.this, "Please enter your password again", Toast.LENGTH_SHORT).show();
} else if (sex<0){
Toast.makeText(RegisterActivity.this, "Please select gender", Toast.LENGTH_SHORT).show();
}else if(!psw.equals(pswAgain)){
Toast.makeText(RegisterActivity.this, "The password entered twice is not the same", Toast.LENGTH_SHORT).show();
/**
* Read the entered username from SharedPreferences and determine if the username is available in SharedPreferences
*/
}else if(isExistUserName(userName)){
Toast.makeText(RegisterActivity.this, "This account name already exists", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(RegisterActivity.this, "Registration successful", Toast.LENGTH_SHORT).show();
//Save the account number, password and account ID to the sp
/**
* Save account number and password to SharedPreferences
*/
saveRegisterInfo(userName, psw);
// pass the account number to LoginActivity.java after successful registration
// return the value to the loginActivity display
Intent data = new Intent();
data.putExtra("userName", userName);
setResult(RESULT_OK, data);
// RESULT_OK is an Activity system constant with a status code of -1.
// indicates that the content operation under this page succeeded in returning the data to the previous page, if it is returned with back then there is no data value passed with setResult
RegisterActivity.this.finish();
}
}
});
}
/**
* Get the string in the control
*/
private void getEditString(){
userName=et_user_name.getText().toString().trim();
psw=et_psw.getText().toString().trim();
pswAgain=et_psw_again.getText().toString().trim();
}
/**
* Read the entered user name from SharedPreferences and determine if the user name is available in SharedPreferences
*/
private boolean isExistUserName(String userName){
boolean has_userName=false;
// mode_private SharedPreferences sp = getSharedPreferences( );
// "loginInfo", MODE_PRIVATE
SharedPreferences sp = getSharedPreferences("loginInfo", MODE_PRIVATE);
//Get the password
String spPsw=sp.getString(userName, "");//pass in the user name to get the password
//If the password is not empty, then the user name was indeed saved
if(!TextUtils.isEmpty(spPsw)) {
has_userName=true;
}
return has_userName;
}
/**
* Save account and password to SharedPreferences SharedPreferences
*/
private void saveRegisterInfo(String userName,String psw){
String md5Psw = MD5Utils.md5(psw);// encrypt the password with MD5
//loginInfo means file name, mode_private SharedPreferences sp = getSharedPreferences( );
SharedPreferences sp=getSharedPreferences("loginInfo", MODE_PRIVATE);
//get the editor, SharedPref
そして最後にパスワード検索画面 activity_lost_find.xml です。
パスワード回復機能は、あくまで新しいアクティビティであり、機能を実装しているわけではありません。
概要:この機能は、内蔵データベースSQLiteを使用せず、MD5暗号化アルゴリズムを使って行われます。
関連
-
Androidでコンストラクタのインテントを解決できない原因と解決策
-
リターンスタックpopBackStack()のAndroidフラグメント、リターンonResumeの問題
-
Android ConstraintLayout コンストレイントレイアウト
-
Android ListViewでaddHeaderを使用する
-
Manifest merger failed : Android 12以降をターゲットとするアプリは、明示的な指定が必要です。
-
Android Studioの設定 Gradleの概要
-
MPAndroidChartのPieChartで、セクターが表示されず、中央のテキストのみが表示される。
-
AndroidのRadioButtonの中央寄せ問題(解決済み)
-
Androidで色を取得するいくつかの方法
-
Android デフォルトのホームアプリケーション(Launcher)起動プロセスのソースコード解析
最新
-
nginxです。[emerg] 0.0.0.0:80 への bind() に失敗しました (98: アドレスは既に使用中です)
-
htmlページでギリシャ文字を使うには
-
ピュアhtml+cssでの要素読み込み効果
-
純粋なhtml + cssで五輪を実現するサンプルコード
-
ナビゲーションバー・ドロップダウンメニューのHTML+CSSサンプルコード
-
タイピング効果を実現するピュアhtml+css
-
htmlの選択ボックスのプレースホルダー作成に関する質問
-
html css3 伸縮しない 画像表示効果
-
トップナビゲーションバーメニュー作成用HTML+CSS
-
html+css 実装 サイバーパンク風ボタン
おすすめ
-
エミュレータです。PANIC: AVDのシステムパスが壊れています。ANDROID_SDK_ROOTの値を確認してください。
-
60フレーム飛ばした!?アプリケーションがメインスレッドで過剰な作業を行っている可能性があります。
-
Android 問題集 No.11:トランスポートエンドポイントが接続されていない
-
Android 高機能版 (xxv) setTextColor() パラメータ設定方法
-
江さんが熟練者から始めさせます。Android Studioは、ランディングページのパスワードスイッチの表示(小さな目)を作成する
-
no target device found 問題が解決した
-
ColorDrawableの簡単な使い方
-
android.content.res.Resources$NotFoundException: 文字列リソースID #0x1
-
android.content.res.Resources$NotFoundException: 文字列リソースID #0x1 Sinkhole!
-
ARMアセンブリ共通命令 NULL演算 NOP命令