std::cinの使用
1. コンセプト
C++の基本的な入出力
C++の標準ライブラリは、豊富な入出力関数を提供しています。ここでは、C++プログラミングで最も基本的で一般的な入出力操作を紹介します。C++の入出力は、バイトのシーケンスであるストリームで行われます。バイトのストリームがデバイス(キーボード、ディスクドライブ、ネットワーク接続など)からメモリに流れる場合、これを「ストリーム」と呼びます。 入力操作 . バイトストリームがメモリからデバイス(ディスプレイ、プリンター、ディスクドライブ、ネットワーク接続など)に流れている場合、これを 出力操作 .
標準出力ストリーム(cout)
coutオブジェクトは、標準出力デバイス(通常はディスプレイ)に接続します。
#include
using namespace std;
int main( )
{
char str[] = "Hello C++";
cout << "Value of str is : " << str << endl;
}
標準入力ストリーム(cin)
定義済みオブジェクト cin は iostream クラスのインスタンスです。cin オブジェクトは標準入力デバイス(通常はキーボード)に接続されます。cin はストリーム抽出演算子 >> と組み合わせて、次のように使用します。
#include
using namespace std;
int main( )
{
char name[50];
cout << "Please enter your name: ";
cin >> name;
cout << "Your name is: " << name << endl;
}
標準エラーストリーム(cerr)
標準的なログストリーム(clog)
参考 http://www.runoob.com/cplusplus/cpp-basic-input-output.html
2. std::cinの共通読み込みメソッド
2.1 cin>>
使用法1:数字を入力する
#include
using namespace std;
int main ()
{
int num;
cout << "Please enter the number: ";
cin >> num;
cout << "The number you entered is: " << num << endl;
}
注意:上記のコードを実行すると、入力が数字以外(例えばa)の場合はcinエラーが発生し、ループで入力した場合はデッドループになります。
使い方2:文字列を受け取り、"space", "TAB" または "enter" で終わらせる。
#include
using namespace std;
int main ()
{
char str[64]={0};
cout << "Please enter a string: ";
cin >> str;
cout << "The string you entered is: " << str << endl;
}
<ブロッククオート
入力:テスト
出力:テスト
Enter: test cin //スペースで終了
出力:テスト
注意: >> は見えない文字(スペース、キャリッジリターン、TABなど)をフィルタリングしているので、ホワイトスペースをスキップしたくない場合は、noskipwsストリームコントロールを使ってください: cin>>noskipws>>str; ここで typing: spaces test は、スペースを出力することになります。
2.2 cin.get()
この関数にはいくつかのオーバーロードがあり、4つの形式があります。パラメータなし、1つの引数、2つの引数、3つの引数です。よく使われる関数のプロトタイプは以下の通りである。
int cin.get();
istream& cin.get(char& var);
istream& get ( char* s, streamsize n );
istream& get ( char* s, streamsize n, char delim );
使い方1:cin.getで文字を読み込む
using namespace std;
int main()
{
char a;
char b;
cout << "Please enter two characters: ";
a=cin.get(); //mode one
cin.get(b); //mode two
cout<<"character a:"<<<a<<"\n character b:"<<b<<endl;
return 0;
}
入力:a [キャリッジリターン]、出力。
注意事項
- 結果からわかるように、cin.get()は入力バッファからセパレータを無視して1文字を直接読み込み、上記のような状況を与え、改行文字を変数bに読み込んで出力に2回出力しています。
- cin.get() の戻り値は int 型で、成功:文字の ASCII 値が読み込まれ、ファイルの終端文字に遭遇すると EOF すなわち -1 が返されます。cin.get(char var) は成功すると cin オブジェクトを返すので cin.get(b).get© のような連鎖操作をサポートすることができます。
使い方2:cin.getは文字列を読み込み、スペースを取ることができる
#include
#include
Input: 123 456 [carriage return], output.
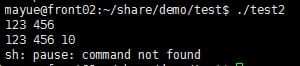
Notes.
As you can see from the results, cin.get(array,20); reads a line and ends the read when it encounters a newline character, but does not process the newline character, which remains in the input buffer. The second time cin.get() reads the newline into variable b, it prints the ASCII value of the input newline as 10. This is the difference between reading a line with cin.get() and reading a line with getline. It also removes '\n' directly from the input buffer and does not affect the following input processing.
cin.get(str,size); reads a line only into a C-style string, i.e. char*, but C++'s getline function can read a string into a C++-style string, i.e. string type. Given these two advantages of getline over cin.get(), the
It is recommended to use getline for line reading.
The usage of getline is described below.
2.3 cin.getline() reads a line
Function: Reads a string from the standard input device keyboard and terminates it with the specified terminator.
There are two function prototypes.
istream& getline(char* s, streamsize count); //default to end with a newline
istream& getline(char* s, streamsize count, char delim);
Use 1.
#include
using namespace std;
int main()
{
char array[20]={0};
// cin.getline(array,20); // or specify the terminator, using the following line
cin.getline(array,20,'\n');
cout<<array<<endl;
return 0;
}
Note that the difference between cin.getline and cin.get is that cin.getline does not leave terminators or newlines in the input buffer.
3. cin needs to enter numbers when entering letters cin status error
Reference.
https://blog.csdn.net/zyb238909/article/details/53056990
https://www.cnblogs.com/tonglingliangyong/p/3908463.html
Example.
#include
#include
using namespace std;
int main( )
{
int num;
while(1)
{
cout << "Please enter the number: ";
cin >> num; //When entering letters or . will cause the cin state to fall into a dead loop
cout << "The number you entered is: " << num << endl;
}
return 0;
}
Note: the above program causes the cin state exception to fall into a dead loop when the letters are entered.
Modified as follows.
#include
#include
#include
using namespace std;
int main( )
{
int num;
while(1)
{
cout << "Please enter the number: ";
cin >> num;
while (cin.fail())
{
cout << "There was an input error! Please retype: ";
cin.clear();
//cin.sync(); // not working well here
cin.ignore(numeric_limits<std::streamsize>::max(),'\n');// clear the current line
// cin.ignore(numeric_limits
4. cin's conditional state
When using cin to read keyboard input, errors are inevitable, and if an error occurs, cin will set the condition state. The condition state identifier is :
goodbit:no error
eofbit:End of file reached
failbit:non-fatal input/output error, reversible
badbit: fatal input/output error, irreversible
If in the input/output class. The iOS::identifier number needs to be added. Corresponding to these conditional states are the member functions of the stream object that set, read, and determine the conditional state. They are mainly.
s.eof(): returns true if the eofbit of stream s is set.
s.fail(): returns true if the failbit of stream s is set.
s.bad(): returns true if the badbit of stream s is set.
s.good(): returns true if the goodbit of stream s is set.
s.clear(flags): clears the status flag bit and sets the given flag bit flags to 1, returning void.
s.setstate(flags): set the corresponding conditional state position in stream s to 1 based on the given flags conditional state flag bit, returning void.
s.rdstate(): return the current conditional state of stream s, return value type strm::iostate. strm::iostate machine-related plastic name, defined by each iostream class, used to define the conditional state.
5. cin emptying the input buffer
cin.clear();
cin.sync();
cin.ignor();
http://blog.sina.com.cn/s/blog_8d3652760100wl9r.html
6. Other ways to read a line of string from standard input
6.1 Reading a line from getline
C++ defines a global function getline in the std namespace, which is declared in the < string> header file because it uses the string as an argument.
getline uses cin to read a line from the standard input device keyboard, and ends the read operation when it 1) reaches the end of the file, 2) encounters the function's delimiter, or 3) reaches the maximum input limit.
The function prototype has two overloaded forms.
istream& getline ( istream& is, string& str);//default ends with a line break
istream& getline ( istream& is, string& str, char delim);
Example usage.
#include
#include
using namespace std;
main ()
{
string str;
getline(cin,str);
cout<<str<<endl;
}
Note: getline() accepts a string that can take spaces and output them.
Note: When getline encounters a terminator, it reads the terminator along with the specified string, and then replaces the terminator with a null character. Therefore, it is safer to use getline when reading a line of characters from the keyboard. However, it is better to perform standard input security checks to improve the program's fault tolerance.
cin.getline() is similar, but cin.getline() is part of the istream stream, while getline() is part of the string stream, which are not the same two functions.
6.2 Getting to read a line
gets is a library function in C, asserted in < stdio.h>, that reads a string from a standard input device, can read infinitely, does not judge the upper limit, and stops reading at the end of a carriage return or EOF, so the programmer should make sure the buffer is large enough so that no overflow occurs when performing a read operation.
Function prototype.
char *gets( char *buffer );
Example usage.
#include <iostream>
#include <stdio.h>
using namespace std;
int main()
{
char array[20]={NULL};
gets(array);
cout<<array<<endl;
return 0;
}
Since this function is a C library function, it is not recommended to use it. Since this is a C++ program, try to use C++ library functions.
Reference.
https://zh.cppreference.com/w/cpp/io/basic_istream
https://blog.csdn.net/bravedence/article/details/77282039
https://www.cnblogs.com/luolizhi/p/5746775.html
istream& getline(char* s, streamsize count); //default to end with a newline
istream& getline(char* s, streamsize count, char delim);
関連
-
c++ プログラミング プロンプトの関数定義はここでは許可されません。
-
C++11での移動セマンティクス(std::move)と完全な前進(std::forward)。
-
解決策:エラー:'cout'は型名ではありません。
-
C++コンパイルエラー:||error: ld returned 1 exit status|.
-
戦闘機ゲームのC++実装(ソースコード)
-
C++ - 文字列クラス超詳細紹介
-
gcc/g++ コンパイル時のエラー解析で期待される型指定子の前に
-
"エラー:不完全なクラス型へのポインタは許可されません。"の前方宣言。
-
C++ shared_ptr コンパイルエラー 'shared_ptr' がこのスコープで宣言されていない問題を修正しました。
-
C/C++アルゴリズムヘッダーファイルのmax(), min(), abs()、数学ヘッダーファイルのfabs()
最新
-
nginxです。[emerg] 0.0.0.0:80 への bind() に失敗しました (98: アドレスは既に使用中です)
-
htmlページでギリシャ文字を使うには
-
ピュアhtml+cssでの要素読み込み効果
-
純粋なhtml + cssで五輪を実現するサンプルコード
-
ナビゲーションバー・ドロップダウンメニューのHTML+CSSサンプルコード
-
タイピング効果を実現するピュアhtml+css
-
htmlの選択ボックスのプレースホルダー作成に関する質問
-
html css3 伸縮しない 画像表示効果
-
トップナビゲーションバーメニュー作成用HTML+CSS
-
html+css 実装 サイバーパンク風ボタン
おすすめ
-
Linux の 'pthread_create' への未定義参照問題を解決しました。
-
C++ std::string は NULL で初期化できない、基本的な使い方
-
C++ JSON ライブラリ jsoncpp 新 API の使用法 (CharReaderBuilder / StreamWriterBuilder)
-
void* から char* への無効な変換」および「文字列定数から 'char*' への非推奨の変換」を解決 "
-
error: '&' トークンの前にイニシャライザーがあるはずです。
-
C++: エラー C2228: '.str' の左側にはクラス/構造体/結合が必要
-
C++ ダイナミックオープンスペース
-
C++はまだデバッグにprintf/coutを使っている、独自のログライブラリの書き方を学ぶ (Previous)
-
const char* から char* へ、const 属性の削除
-
vs2010 エラー: エラー LNK1123: COFF への変換に失敗しました: ファイルが無効か破損しています。